Table Of Content
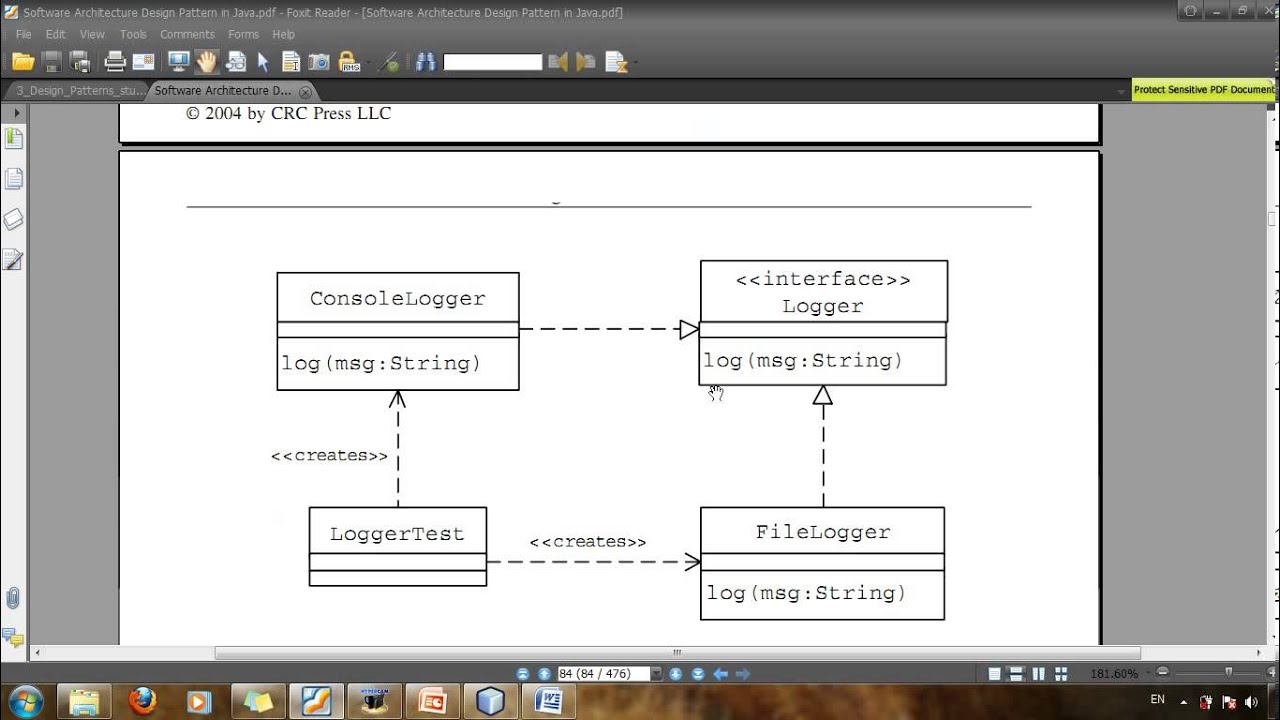
So far we learned what is Factory method design pattern and how to implement it. I believe now we have a fair understanding of the advantage of this design mechanism. Factory methods pervade toolkits and frameworks.The preceding document example is a typical use in MacApp and ET++. Adapter Method is a structural design pattern, it allows you to make two incompatible interfaces work together by creating a bridge between them. The Data Access Object (DAO) design pattern is used to decouple the data persistence logic to a separate layer.
2 Abstract Factory Method
Now that we have super classes and sub-classes ready, we can write our factory class. Lets you define a family of algorithms, put each of them into a separate class, and make their objects interchangeable. Lets you produce families of related objects without specifying their concrete classes. A design pattern is a generic repeatable solution to a frequently occurring problem in software design that is used in software engineering.
Important Points to Keep in Mind for the Factory Method in Java Design Patterns
Having said that I want to invite you to partner me in clearing the space and providing the correct Design patterns as they are and not as they occur to you, me or any other author. I want to point out that the example you have put is neither of that. In the factory pattern , the factory class has an abstract method to create the product and lets the sub classes to create the concrete product.
Product
In the string conversion example, we demonstrated how the Factory Design Pattern allows us to switch between different conversion types easily. This pattern promotes modularity, extensibility, and code reusability, making it a valuable tool in software development. Abstract Factory Method is a creational design pattern, it provides an interface for creating families of related or dependent objects without specifying their concrete classes.
DZone Coding Java The Factory Pattern Using Lambda Expressions in Java 8 - DZone
DZone Coding Java The Factory Pattern Using Lambda Expressions in Java 8.
Posted: Wed, 01 Mar 2017 08:00:00 GMT [source]
As the initial requirements were just to build a simple SMS notifications class that contains business logic along with other information. Enumeration below represents types of coins that we support (GoldCoin and CopperCoin). Buy the eBook Dive Into Design Patterns and get the access to archive with dozens of detailed examples that can be opened right in your IDE. Use the Factory Method when you want to provide users of your library or framework with a way to extend its internal components.
In the example, INRFactory, USDFactory, and GBPFactory are concrete factory implementations. When the factory method comes into play, you don’t need to rewrite the logic of the Dialog class for each operating system. If we declare a factory method that produces buttons inside the base Dialog class, we can later create a subclass that returns Windows-styled buttons from the factory method. The subclass then inherits most of the code from the base class, but, thanks to the factory method, can render Windows-looking buttons on the screen. As long as all product classes implement a common interface, you can pass their objects to the client code without breaking it. If object creation code is spread in the whole application, and if you need to change the process of object creation then you need to go in each and every place to make necessary changes.
Then we have the static method getCoin to create coin objects encapsulated in the factory class CoinFactory. Factory is an object for creating other objects – formally a factory is a function or method that returns objects of a varying prototype or class. It's a misconception that design patterns are holy solutions to all problems. Design patterns are techniques to help mitigate some common problems, invented by people who have solved these problems numerous times.
Implementation
If you still have some doubt on abstract factory design pattern in Java, please leave a comment. CarType will hold the types of car and will provide car types to all other classes. Defines the skeleton of an algorithm in the superclass but lets subclasses override specific steps of the algorithm without changing its structure. Mediator Method is a Behavioral Design Pattern, it promotes loose coupling between objects by centralizing their communication through a mediator object. Instead of objects directly communicating with each other, they communicate through the mediator, which encapsulates the interaction and coordination logic.
The adapter design pattern is one of the structural design patterns and is used so that two unrelated interfaces can work together. The object that joins these unrelated interfaces is called an adapter. The abstract factory defines an interface for creating buttons and checkboxes. There are two concrete factories, which return both products in a single variant.
To solve this problem, we can create a Factory of spaceships and leave the specifics (which engine or dish is used) to the subclasses to define. The client can then create objects through a common interface which simplifies the process. This is an interface or an abstract class that declares the method for creating objects. In the example, CurrencyFactory is the factory interface with the method createCurrency().
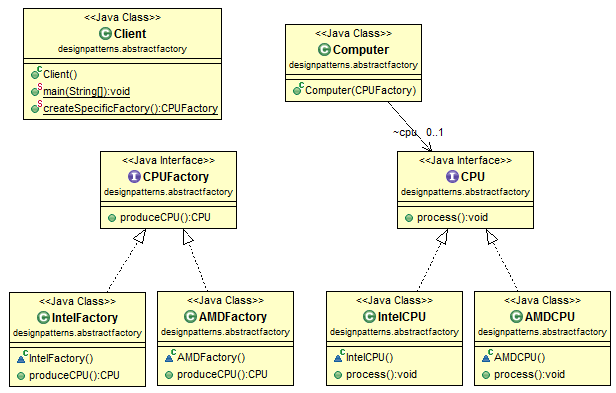
It is one of the best ways to create an object where object creation logic is hidden from the client. Factory method is a creational design pattern which solves the problem of creating product objects without specifying their concrete classes. Pankaj, You have a wide reach and your article make a huge impact on developers. I appreciate your work and dedication that you put to bring this in front of us all.
This approach separates the object creation from the implementation, which promotes loose coupling and thus easier maintenance and upgrades. For example, to add a new product type to the app, you’ll only need to create a new creator subclass and override the factory method in it. As you can see, the factory is able to return any type of car instance it is requested for. It will help us in making any kind of changes in car making process without even touching the composing classes i.e. classes using CarFactory.
The client code calls the creation methods of a factory object instead of creating products directly with a constructor call (new operator). Since a factory corresponds to a single product variant, all its products will be compatible. This type of design pattern comes under creational pattern as this pattern provides one of the best ways to create an object.
No comments:
Post a Comment